Firebase
Firebase๋ ๊ณ ํ์ง ์ฑ์ ๋น ๋ฅด๊ฒ ๊ฐ๋ฐํ๊ณ ๋น์ฆ๋์ค๋ฅผ ์ฑ์ฅ์ํค๋ ๋ฐ ๋์์ด ๋๋ Google์ ๋ชจ๋ฐ์ผ ํ๋ซํผ์ ๋๋ค.
firebase.google.com
1. npm install
https://www.npmjs.com/package/firebase
npm install --save firebase
2. firebase ์ด๊ธฐํ
Firebase > Console > ํ๋ก์ ํธ ์์ฑ > ํ๋ก์ ํธ > ํ๋ก์ ํธ ์ค์
์๋์ Firebase SDK ๋ฅผ ๋ณต์ฌํด ์ค๋๋ค.
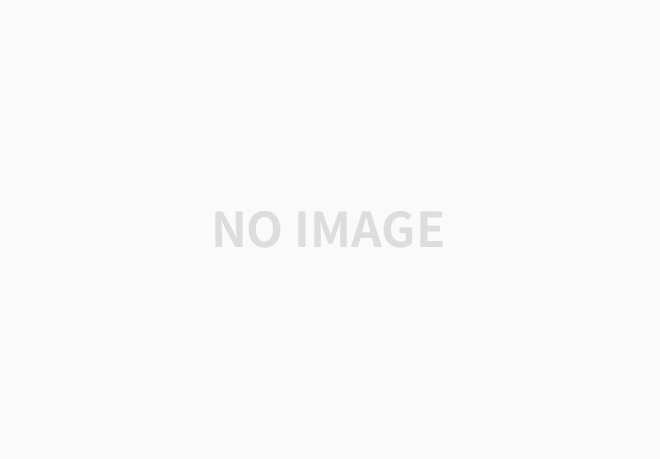
Firebase์ ๊ด๋ จ๋ ์๋น์ค๋ค์ ์ปดํฌ๋ํธ์ ๋ฐ๋ก ๋ง๋ค๊ธฐ ์ํด, srcํด๋์ service ํด๋๋ฅผ ์์ฑ ํ firebase.js ์์ฑํด ์ค๋๋ค.
../src/service/firebase.js
๋ณต์ฌํ ๋ด์ฉ์ ๋ถ์ฌ์ค๋๋ค.
- ํ์ ์๋ ๊ฒ๋ค์ ์ ์จ์ฃผ๊ณ , ๋ก๊ทธ์ธ๊ณผ ํ์๊ฐ์ ํ ๋ ํ์ํ api key์ auth domain๋ง ๋จ๊ฒจ์คฌ์ต๋๋ค.
- key๋ ์ธ๋ถ์ ๋ ธ์ถ๋๋ฉด ์ ๋๋๊น, env ํ์ผ์ ์จ๊ฒจ์ค๋๋ค.
import { initializeApp } from "firebase/app";
import { getAuth } from "firebase/auth";
/** ํ๋ก์ ํธ config */
const firebaseConfig = {
apiKey: process.env.REACT_APP_FIREBASE_API_KEY,
authDomain: process.env.REACT_APP_FIREBASE_AUTH_DOMAIN,
};
// Initialize Firebase
const app = initializeApp(firebaseConfig);
// Authentication SDK ์ถ๊ฐ ๋ฐ ์ด๊ธฐํ
// Initialize Firebase Authentication and get a reference to the service
const auth = getAuth(app);
export { auth };
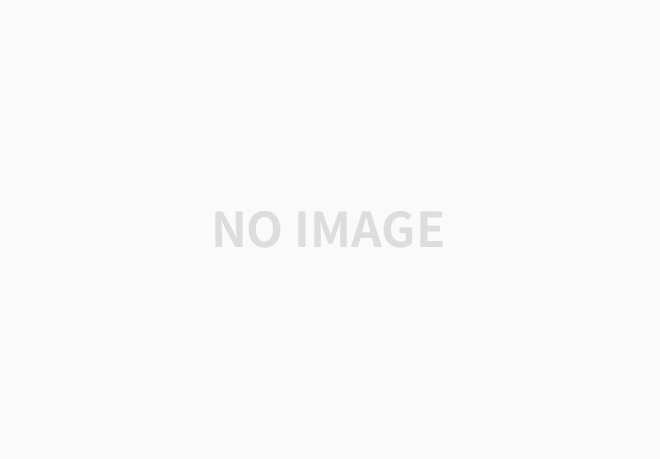
๋ก๊ทธ์ธ์ ํ ๋, ์ด๋ ๊ฒ Google ๋ก๊ทธ์ธ ํ์ ์ฐฝ์ด ๋จ๋๋ก ํด๋ณด๊ฒ ์ต๋๋ค!
3. Google ๋ก๊ทธ์ธ ์ ์ฒด ์ค์
์ฐ์ , ์ฐ๊ฒฐํ๊ณ ์ถ์ ๋ก๊ทธ์ธ ์ ์ฒด๋ฅผ ์ถ๊ฐํด์ฃผ์ธ์. (์ ๋ Google๋ง ํ์ต๋๋ค.)
Firebase > Console > ๋ณธ์ธ project ์ ํ > Authentication > Sign-in method
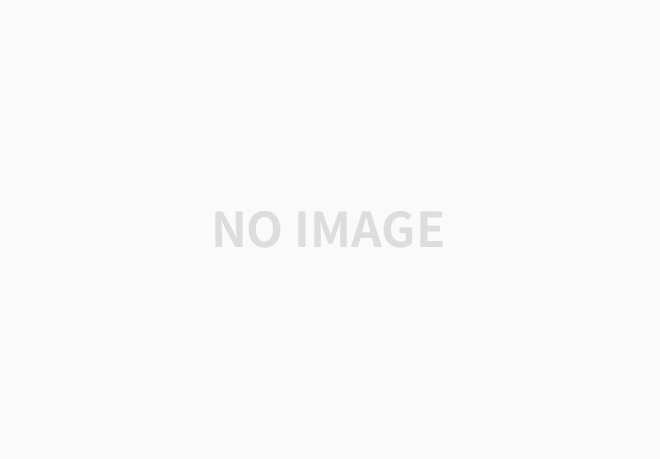
4. Google ์ฌ์ฉํ์ฌ ์ธ์ฆ
https://firebase.google.com/docs/auth/web/google-signin?authuser=0
์๋ฐ์คํฌ๋ฆฝํธ๋ก Google์ ์ฌ์ฉํ์ฌ ์ธ์ฆ | Firebase
Google์ ํ์ธ ๊ณต๋์ฒด๋ฅผ ์ํ ์ธ์ข ์ ํ๋ฑ์ ์ถ๊ตฌํ๊ธฐ ์ํด ๋ ธ๋ ฅํ๊ณ ์์ต๋๋ค. ์์ธํ ์์๋ณด๊ธฐ ์๊ฒฌ ๋ณด๋ด๊ธฐ ์๋ฐ์คํฌ๋ฆฝํธ๋ก Google์ ์ฌ์ฉํ์ฌ ์ธ์ฆ ์ปฌ๋ ์ ์ ์ฌ์ฉํด ์ ๋ฆฌํ๊ธฐ ๋ด ํ๊ฒฝ์ค์ ์ ๊ธฐ
firebase.google.com
../src/service/firebase.js ์ Google auth provider๋ฅผ ์ถ๊ฐํด์ค๋๋ค.
import { initializeApp } from "firebase/app";
import { getAuth, GoogleAuthProvider } from "firebase/auth";
/** ํ๋ก์ ํธ config */
const firebaseConfig = {
apiKey: env.REACT_APP_FIREBASE_API_KEY,
authDomain: env.REACT_APP_FIREBASE_AUTH_DOMAIN,
};
// Initialize Firebase
const app = initializeApp(firebaseConfig);
// Authentication SDK ์ถ๊ฐ ๋ฐ ์ด๊ธฐํ
// Initialize Firebase Authentication and get a reference to the service
const auth = getAuth(app);
/** ๊ตฌ๊ธ auth provider */
const googleAuthProvider = new GoogleAuthProvider();
export { auth, googleAuthProvider };
../src/service/auth.js ์ ์์ฑํด์ฃผ๊ณ , ํด๋น ์ฝ๋๋ฅผ ์์ฑํ์ฌ ๋ก๊ทธ์ธ ํ์ ์ฐฝ์ ๊ตฌํํด์ค๋๋ค.
import { signInWithPopup, GoogleAuthProvider } from "firebase/auth";
import { auth, googleAuthProvider } from "./firebase";
/** ๋ก๊ทธ์ธ ํ์
*/
export const signInGoogle = signInWithPopup(auth, googleAuthProvider)
.then((result) => {
// This gives you a Google Access Token. You can use it to access the Google API.
const credential = GoogleAuthProvider.credentialFromResult(result);
const token = credential.accessToken;
// The signed-in user info.
const user = result.user;
// ...
})
.catch((error) => {
// Handle Errors here.
const errorCode = error.code;
const errorMessage = error.message;
// The email of the user's account used.
const email = error.customData.email;
// The AuthCredential type that was used.
const credential = GoogleAuthProvider.credentialFromError(error);
// ...
});
signInGoogle์ ์ ํ์ฉํ์ฌ, ๋ณธ์ธ์ ํ๋ก์ ํธ ๋ด์์ ๋ก๊ทธ์ธ ํ์ ์ฐฝ์ ๋จ๊ฒ ํ ๊ณณ์ ์ฐ๊ฒฐํฉ๋๋ค.
์๋ฅผ ๋ค์ด, ๋ฒํผ์ ํด๋ฆญํ๋ฉด Google ๋ก๊ทธ์ธ ํ์ ์ฐฝ์ด ๋จ๋๋ก...